Did you know that caching can reduce application response times by up to 90%? This powerful technique helps you retrieve data faster, ensuring your users get the speed they expect. Whether you’re building a small app or scaling a massive platform, understanding how to implement caching can make a world of difference.
At its core, caching allows you to store frequently accessed datum in a temporary location. This avoids repetitive queries to your primary data source, cutting down on latency. For instance, instead of running the same SQL statement multiple times, you can retrieve the ResultSet data once and store it efficiently using tools like Redis.
In this guide, we’ll explore various strategies like cache-aside, read-through, and write-through. These methods help bridge the gap between slow data sources and the need for quick responses in your application. By the end, you’ll have a clear, step-by-step understanding of how to implement these techniques effectively.
Introduction to Database Caching
Ever wondered how to speed up your app without overloading your data source? Caching is the answer. It’s a temporary storage layer that keeps frequently accessed data ready for quick retrieval. This approach cuts down on repetitive queries, making your app faster and more responsive.
By storing data in a high-speed layer, you reduce the load on your primary storage. This improves system performance and ensures smoother operations. Think of it as having a shortcut to your most-used information, saving both time and resources.
One key feature of caching is the time-to-live (TTL) setting. This determines how long data stays in the cache before it’s refreshed. Consistent access models also play a role in maintaining a healthy cache, ensuring data remains accurate and up-to-date.
In relational databases, caching can significantly enhance read speeds. For example, instead of querying the database repeatedly, you can fetch data once and store it for future use. This not only boosts performance but also reduces latency, making your app more efficient.
As you dive deeper into caching, you’ll discover its many benefits. From improving access speeds to reducing the load on your primary storage, it’s a game-changer for modern applications. Stay tuned as we explore more strategies in the next sections.
Understanding the Benefits of Database Caching
What if your app could respond to user requests in milliseconds? By storing frequently accessed data in a temporary layer, you can achieve this level of speed. This approach reduces latency, ensuring your app feels snappy and responsive.
One of the biggest advantages is the reduction in load on your primary storage. Instead of querying the same data repeatedly, you can retrieve it once and store it for future use. This not only speeds up request times but also frees up resources for other tasks.
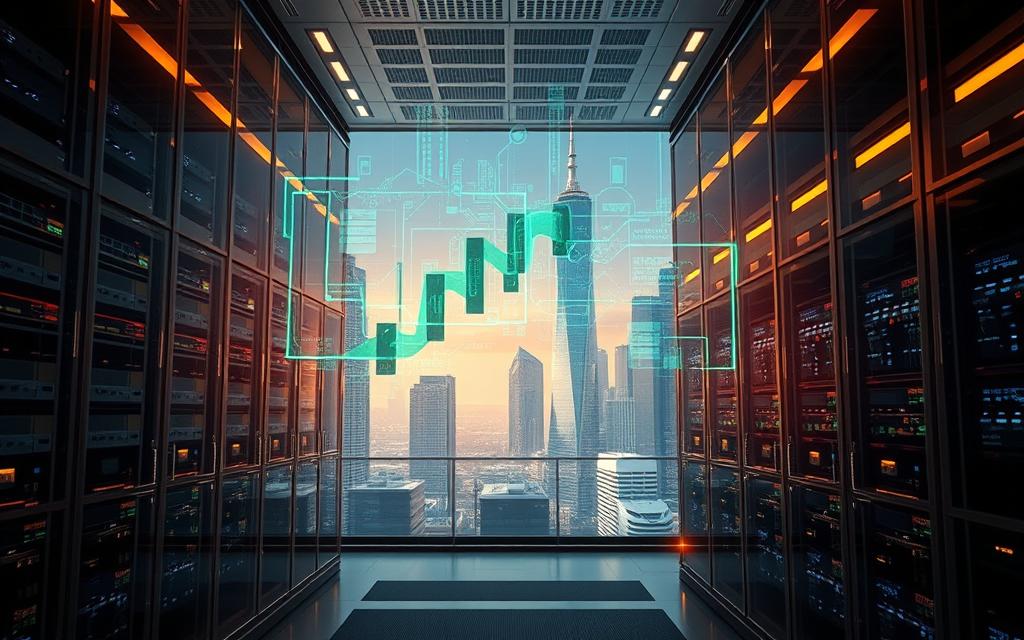
Another key benefit is improved memory utilization. By caching common queries, you ensure that only essential data is stored. This optimizes your system’s performance and reduces unnecessary overhead.
During peak traffic, caching can be a lifesaver. It helps your app handle more requests without slowing down. This leads to a smoother user experience and prevents crashes during high load periods.
Consistency is also a major advantage. By integrating caching into your storage strategy, you ensure that data remains accurate and up-to-date. This builds trust with your users and enhances overall system stability.
Benefit | Impact |
---|---|
Reduced Latency | Faster response times for user requests |
Lower Load | Decreased pressure on primary storage |
Memory Optimization | Efficient use of system resources |
Peak Traffic Handling | Improved performance during high load periods |
Data Consistency | Accurate and up-to-date information for users |
Exploring Various Caching Strategies
Want faster data access? The right caching approach makes it happen. Different strategies suit different needs, and choosing the best one can significantly improve your app’s performance. Let’s dive into the most effective methods and when to use them.
Cache-aside Method Explained
The cache-aside method is simple and widely used. Here’s how it works: your app checks the cache first for the requested data. If it’s not there, the app fetches it from the primary storage and stores it in the cache for future use.
This approach is ideal for lazy loading, where data is only retrieved when needed. For example, in SQL-based apps, you can avoid repetitive queries by storing the ResultSet data in the cache after the first fetch.
Comparing Read-through and Write-through Approaches
Read-through and write-through methods automate data synchronization. In read-through, the cache fetches data from storage if it’s not already cached. Write-through ensures data is written to both the cache and storage simultaneously.
Read-through is great for read-heavy operations, while write-through maintains consistency. However, write-through can introduce latency due to dual writes. Libraries often handle these processes, making them easier to implement.
Delving into Write-back and Write-around Techniques
Write-back and write-around techniques focus on optimizing write operations. Write-back stores data in the cache first and writes it to storage later, improving speed but risking data loss if the cache fails.
Write-around bypasses the cache entirely for writes, reducing cache pollution. This is useful for data that’s rarely read. Both methods have their trade-offs, so choose based on your app’s needs.
Strategy | Best Use Case | Pros | Cons |
---|---|---|---|
Cache-aside | Lazy loading | Simple, reduces repetitive queries | Manual cache management |
Read-through | Read-heavy operations | Automated, consistent | Can introduce latency |
Write-through | Data consistency | Ensures accuracy | Slower writes |
Write-back | Write optimization | Faster writes | Risk of data loss |
Write-around | Infrequently read data | Reduces cache pollution | May increase read latency |
Each strategy impacts your app’s operations differently. Whether you prioritize speed, consistency, or storage efficiency, understanding these methods ensures you make the right choice for your needs.
Practical Steps to Implement Database Caching Mechanisms
Ready to optimize your app’s performance with practical steps? This section walks you through the process of implementing effective caching techniques. You’ll learn how to handle SQL ResultSet data and leverage Redis for high-speed storage.
Caching SQL ResultSet Data
Start by executing your SQL query and retrieving the ResultSet. Instead of querying the database repeatedly, store the data in a temporary layer. This reduces the workload on your primary storage and speeds up access times.
To cache the ResultSet, serialize it into a byte array using a CachedRowSet object. This allows you to store the data efficiently. Here’s a simple example in Java:
CachedRowSet crs = RowSetProvider.newFactory().createCachedRowSet(); crs.populate(resultSet); ByteArrayOutputStream bos = new ByteArrayOutputStream(); ObjectOutputStream oos = new ObjectOutputStream(bos); oos.writeObject(crs);
Set a Time-to-Live (TTL) for the cached data to ensure it stays fresh. This technique ensures that your app retrieves the most up-to-date information while minimizing resource usage.
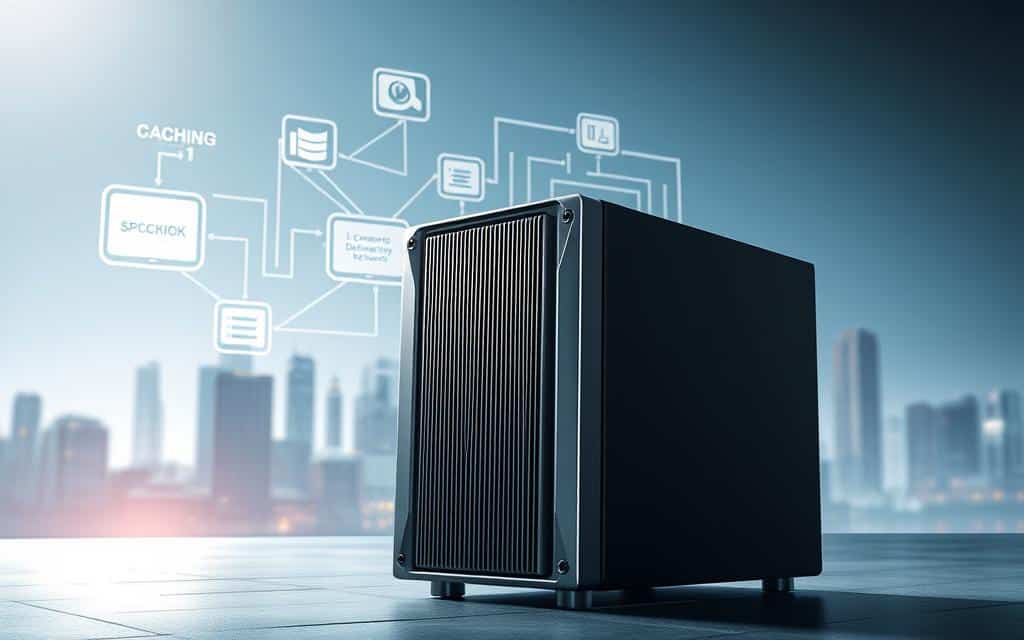
Leveraging Redis for Effective Caching
Redis is a powerful tool for storing frequently accessed data. Start by setting up a Redis client like Jedis in your application. This allows you to handle caching tasks seamlessly.
Use the following steps to store your serialized ResultSet in Redis:
- Connect to your Redis instance using the Jedis client.
- Store the byte array with a unique key, such as “resultset:query123”.
- Set a TTL for the key to manage data availability.
Here’s an example of how to do this in Java:
Jedis jedis = new Jedis("localhost"); jedis.set("resultset:query123".getBytes(), bos.toByteArray()); jedis.expire("resultset:query123", 3600); // TTL of 1 hour
This approach ensures that your app retrieves data quickly, reducing latency and improving performance.
By following these steps, you can significantly reduce the workload on your primary storage. This leads to faster response times and better resource utilization. Plus, your app will remain highly available even during peak traffic.
If you encounter issues, double-check your Redis connection and ensure your serialization process is error-free. These troubleshooting tips will help you maintain smooth operations.
In summary, these techniques balance performance with efficient resource usage. Whether you’re caching SQL ResultSet data or leveraging Redis, these steps ensure your app runs smoothly and efficiently.
Leveraging Caching to Boost Application Performance
Looking to supercharge your app’s speed? Redis is your go-to tool for making it happen. By storing frequently accessed data in memory, Redis reduces response times and takes the load off your server. This not only improves performance but also lowers operational cost.
One of the biggest benefits of Redis is its ability to handle high traffic without slowing down. For example, in-memory storage can retrieve data up to 100 times faster than traditional disk-based systems. This means your app stays responsive even during peak usage.
Effective key management is another way to optimize performance. Use clear naming conventions like “user:123:profile” to simplify cache retrieval. This makes it easier to locate and update data, ensuring your app runs smoothly.
Here are some practical tips to get the most out of Redis:
- Set a Time-to-Live (TTL) for each key to keep data fresh.
- Use Redis clustering to distribute the load across multiple servers.
- Monitor cache hit rates to ensure your system is performing efficiently.
By integrating Redis into your workflow, you’ll see significant improvements in speed and resource utilization. For instance, studies show that caching can reduce server bandwidth usage by up to 60%, leading to substantial cost savings.
Balancing performance and cost is key. While Redis offers incredible speed, it’s important to secure your cache to protect sensitive data. Use encryption and access controls to keep your information safe.
In summary, Redis and smart key management can transform your app’s performance. Faster response times, lower costs, and improved server efficiency are just a few of the benefits you’ll enjoy. Start implementing these strategies today and watch your app thrive.
Overcoming Common Caching Challenges
Caching can be a game-changer, but it’s not without its hurdles. From stale data to synchronization issues, these challenges can impact your app’s performance. Let’s explore how to tackle them effectively.
Ensuring Data Consistency and Synchronization
One of the biggest challenges is keeping your cache in sync with the primary database. Stale data can lead to incorrect results, frustrating users and harming your app’s reliability. To avoid this, use Time-to-Live (TTL) settings to refresh data regularly.
Synchronization is equally important. When updates occur, ensure they’re reflected in both the cache and the primary database. Techniques like write-through and write-behind can help maintain consistency across systems.
Strategies for Efficient Cache Invalidation
Cache invalidation ensures outdated data is removed promptly. Explicit invalidation routines can help, but they require careful planning. For example, when a query updates a record, invalidate the corresponding cache entry immediately.
Another approach is to use eviction policies like LRU (Least Recently Used). This removes less frequently accessed data, freeing up space for fresh entries. Randomizing TTL values can also prevent simultaneous expirations, reducing the risk of cache stampedes.
Challenge | Solution |
---|---|
Stale Data | Use TTL settings and explicit invalidation |
Inconsistency | Implement write-through or write-behind techniques |
Cache Stampedes | Randomize TTL values and use eviction policies |
These strategies not only improve application performance but also enhance scalability. By addressing these challenges, you can ensure your app remains fast and reliable, even as it grows.
Best Practices and Strategic Tips for Optimized Caching
Ever thought about how to keep your app running smoothly under heavy load? The answer lies in smart strategies and best practices. By focusing on key naming conventions, TTL management, and maintaining consistency, you can ensure your app stays fast and reliable.
Key Naming Conventions and TTL Management
Clear and predictable key names make it easier to manage your system. Use formats like “object:type:id” to simplify retrieval and maintenance. For example, “user:123:profile” clearly identifies the data type and its purpose.
Setting appropriate TTL values is another critical step. It ensures your data stays fresh while reducing the load on your primary storage. For instance, a TTL of 3600 seconds (1 hour) works well for frequently updated information.
Ensuring Consistency and Handling Retrieval Challenges
Keeping your cache in sync with the primary storage is a common challenge. Techniques like write-through and write-behind help maintain consistency. For example, write-through ensures data is updated in both the cache and storage simultaneously.
Monitoring retrieval performance is equally important. Track cache hits and misses to identify inefficiencies. If you notice frequent misses, adjust your TTL values or review your key naming conventions.
Strategic Tips for Overcoming Common Challenges
Here are some actionable tips to keep your caching strategy robust:
- Use eviction policies like LRU (Least Recently Used) to free up space for fresh entries.
- Randomize TTL values to prevent simultaneous expirations and cache stampedes.
- Monitor metrics like latency and memory usage to detect and address issues early.
By adopting these best practices, you can ensure your app remains fast, efficient, and scalable. Start implementing these strategies today and see the difference they make.
How Does Database Replication Impact Caching Performance?
Efficient caching relies on consistent data, but database replication can introduce complexities. With database replication methods explained clearly, it’s easier to understand how replication lag affects cache freshness. Synchronized replication enhances cache performance, while delays may cause stale data, requiring smart invalidation strategies to maintain accuracy and system efficiency.
Wrapping Up Your Database Caching Journey
By now, you’ve seen how strategic storage techniques can transform your app’s efficiency. Implementing these methods boosts speed, reduces response times, and ensures smoother operations. Whether you’re handling a small project or scaling a large platform, the right approach makes all the difference.
From cache-aside to write-through, each strategy has its pros and cons. For example, cache-aside is simple but requires manual management, while write-through ensures consistency but may slow down writes. Choosing the right method depends on your app’s needs.
Continuous monitoring and adjustments are key. Regularly check metrics like cache hits and misses to maintain optimal performance. Small changes can lead to significant improvements over time.
Take these insights and apply them to your own challenges. Experiment with different techniques, monitor the results, and refine your strategy. Your app’s performance will thank you.
Ready to dive deeper? Explore more ways to optimize your system and keep your app running at its best.